When writing a program in JavaScript, the process of filtering the elements that satisfy the conditions from the array frequently occurs.
In this article, I will explain the filter
function, which makes it easier to write such a process than using a for
or if
statement!
By the end of this article, you will be able to learn the filter
function, which is useful for filter array elements. And, compared to the case of using the for
and if
statement, you will be able to perform array operations with a very concise way.
Table of Contents(目次)
Target audience
I’m writing this article for JavaScript beginners.
I think this article is useful for those who use for
and if
statements when filtering array elements.
In this article, lambda expression appears in the text. (e.g. () => console.log('Hello world!')
)
This article can be read by those who do not know lambda expressions, but using lambda expressions has a great advantage because it makes it possible to write functions in a short and concise way.
The following article explains lambda expression for beginners. If you don’t know about it, please take a look if you like. You can read it in about 5 minutes.
When filtering elements using for and if statements
At first, in order to compare with the case of using the filter
function, we write the element filtering process using the for
and if
statement.
As an example, let’s write a process to extract only even elements from a number array. It will be as follows.
let numList = [ 1, 2, 3, 4, 5 ]
// using if and for statement
let resultList = []
for (let i=0; i<numList.length; i++) {
if (numList[i] % 2 === 0) {
resultList.push(numList[i])
}
}
If you use for
and if
statement, you need to declare an array to store the filtering results. In this example, it is resultList
.
Then, check if each element meets the conditions. If you find one that meets the conditions, push it to the resulting array.
When using the filter function
The filter
function is a convenient function for extracting only the elements that meet the conditions from the array.
As a usage, if you use it like array.filter(extraction_function)
, only the elements whose return value of the extraction_function
is true will be extracted.
It is difficult to understand the behavior only by words alone, so let’s take a look at the process of extracting only even-numbered elements as an example.
When you execute the following code, the variable resultList
will contain an array ([2, 4]
) with only even numbers extracted.
let numList = [ 1, 2, 3, 4, 5]
// using filter function to extract even-numbered elements
let resultList = numList.filter(function (e) {
return e % 2 === 0
})
Let’s walk through this code step by step.
First, the extraction_function
in the above example is the function like below.
function (e) {
return e % 2 === 0
}
This function takes the argument e
and returns true if it is even.
In short, the filter
function is a function that passes each element of the array in order in the argument e of this extraction_function
and calls it, then leaving only the element whose result is true!
Below is a figure of the behavior of the filter
function. The dotted line element on the right side of the figure is not included in the resultList
because the result of the extraction_function
is false. The solid line element is included in the resultList
because the result of the extraction_function
is true.
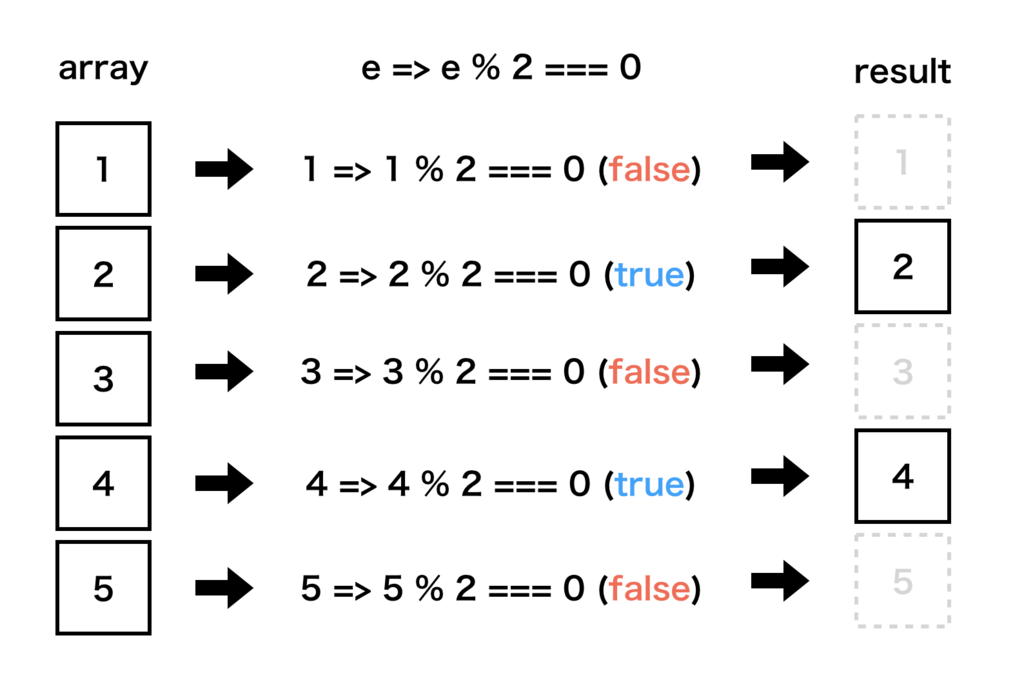
Also, unlike the case of using the for
/ if
statement, you do not need to prepare an array for the result yourself. The filter function internally prepares a new array and returns it.
Compared to the case using for
and if
statement, you can see that it can be written shorter by using the filter
function.
let numList = [ 1, 2, 3, 4, 5]
// using for and if statement
let resultList = []
for (let i=0; i<numList.length; i++) {
if (numList[i] % 2 === 0) {
resultList.push(numList[i])
}
}
// using filter function
let resultList = numList.filter(function (e) {
return e % 2 === 0
})
By the way, you can change the name of the argument of extraction_function
as you like. In the examples so far, we have named it e
, which is the first character of the word “element”. However, for example, you can name the argument num
to make it easier to see that it is a number.
// you can change the name of arguments
let resultList = numList.filter(function (num) {
return num % 2 === 0
})
Write a filter function more concisely using lambda expression
We found it very easy to extract elements using the filter function. But you can write it more concisely by using the lambda expression.
let numList = [ 1, 2, 3, 4, 5]
// using for and if statement
let resultList = []
for (let i=0; i<numList.length; i++) {
if (numList[i] % 2 === 0) {
resultList.push(numList[i])
}
}
// using filter function without lambda expression
let resultList = numList.filter(function (e) {
return e % 2 === 0
})
// using filter function with lambda expression
let resultList = numList.filter(e => e % 2 === 0)
If you use a lambda expression, it will be very intuitive because you only have to think about the judgment expression that the element you want to keep will be true and the element you do not want to keep will be false. And write the judgment expression on the right side of the arrow.
I won’t go into detail about lambda expressions in this article. If you want to know more about the lambda expression, please read the following article if you have time.
Some examples that using filter function
Let’s look at other examples to get a deeper understanding of how to use it.
Example 1: Extract only odd numbers
let numList = [ 1, 2, 3, 4, 5]
// using for and if statement
let resultList = []
for (let i=0; i<numList.length; i++) {
if (numList[i] % 2 === 1) {
resultList.push(numList[i])
}
}
// using filter function without lambda expression
let resultList = numList.filter(function (e) {
return e % 2 === 1
})
// using filter function with lambda expression
let resultList = numList.filter(e => e % 2 === 1)
Example 2: Extract only names starting with ‘J’
let nameList = [
'John Smith',
'David Brown',
'James Moore'
]
// using for and if statement
let resultList = []
for (let i=0; i<nameList.length; i++) {
if (nameList[i].startsWith('J')) {
resultList.push(nameList[i])
}
}
// using filter function without lambda expression
let resultList = nameList.filter(function (name) {
return name.startsWith('J')
})
// using filter function with lambda expression
let result = nameList.filter(name => name.startsWith('J'))
Example 3: Extract only people in their 20s
let users = [
{ name: 'John' , age: 25 },
{ name: 'David' , age: 27 },
{ name: 'James' , age: 31 },
{ name: 'Richard', age: 21 }
]
// using for and if statement
let resultList = []
for (let i=0; i<users.length; i++) {
if (users[i].age >= 20 && users[i].age < 30) {
resultList.push(users[i])
}
}
// using filter function without lambda expression
let resultList = users.filter(function (user) {
return user.age >= 20 && user.age < 30
})
// using filter function with lambda expression
let result = users.filter(user => user.age >= 20 && user.age < 30)
In case you want to use element index to filter
As explained above, the filter
function is used like array.filter(extraction_function)
.
If you want to use an element position in your extraction condition (e.g. if you want to extract even-numbered elements), you can use the second argument of extraction_function
.
Second argument is a position of the element.
The following is an example of extracting only even-numbered elements.
Note that the element numbers start at 0. The element number (= variable i
in this example) is 0,1,2,3 …. If the remainder of dividing the element number by 2 is 1, the element is even.
let numList = [ 1, 2, 3, 4, 5 ]
// using for and if statement
let resultList = []
for (let i=0; i<numList.length; i++) {
if (i % 2 == 1) {
resultList.push(numList[i])
}
}
// using filter function without lambda expression
// i is element position
let resultList = numList.filter(function (e, i) {
return i % 2 === 1
})
// using filter function witho lambda expression
// i is element position
let resultList = numList.filter((e, i) => i % 2 === 1)
If you do not use the element position as a condition, you can omit the second argument.
Achieve complex filtering by chaining filter functions
Since the filter
function returns an array as the return value, you can write this function continuously.
Calling multiple methods in succession in this way is called a method chain. This is because the methods are continuous like a chain.
Sometimes code is intuitive and readable if you chain multiple filter
function rather than specifying complicated conditions with one filter
.
The following example extracts a female in her twenties from the list of users. It is readable, isn’t it?
let users = [
{ name: 'David', gender: 'Man', age: 25 },
{ name: 'Olivia', gender: 'Woman', age: 27 },
{ name: 'Emma', gender: 'Woman', age: 31 },
{ name: 'Isabella', gender: 'Woman', age: 21 }
]
let result = users.filter(user => user.gender === 'Woman')
.filter(user => user.age >= 20)
.filter(user => user.age < 30)
Conclusion
In this article, I introduced the filter
function, which allows you to briefly write the process of extracting the elements of an array that meet the conditions.
Before you get used to it, you may be confused by the difference from the method using for
and if
statements. However, once you get used to it, you can write intuitive code with a small number of lines.
Please practice so that you can master it!