Hello! I’m Mido, an indie game developer. You can follow me on Twitter! (@mido_app)
I imagine many indie developers create custom utility libraries to improve their development efficiency by extracting frequently used processes.
In this article, I will introduce a utility class that I found useful for calculating random numbers and setting ranges easily.
Table of Contents(目次)
Implementation of the Random Number Utility Class (Integer Version)
Let’s dive into the implementation!
Below is a utility class that allows you to easily obtain random integers between a specified minimum and maximum range.
using System;
using UnityEngine;
[Serializable]
public class IntRange
{
[SerializeField]
private int _min;
public int Min
{
get { return _min; }
set { _min = value; }
}
[SerializeField]
private int _max;
public int Max
{
get { return _max; }
set { _max = value; }
}
public int GetRandomValueInRange()
{
return UnityEngine.Random.Range(Min, Max);
}
}
It’s a very simple implementation, but by adding the [Serializable]
attribute, you can set the values in the Unity Inspector, which allows you to adjust the balance while the game is running. A favorite feature of mine!
Also, note that with UnityEngine.Random.Range
, the minimum value is inclusive, but the maximum value is exclusive. So if Min is 0 and Max is 10, the random number will be between 0 <= x <= 9
.
Example Usage of the Random Number Utility Class
Using this class is very simple. You can define a field in your MonoBehaviour script as follows:
using UnityEngine;
public class Enemy : MonoBehaviour
{
[SerializeField]
private IntRange _maxHpRange;
[SerializeField]
private IntRange _attackRange;
private int _maxHp;
public int MaxHp => _maxHp;
private int _attack;
public int Attack => _attack;
void Start()
{
_maxHp = _maxHpRange.GetRandomValueInRange();
_attack = _attackRange.GetRandomValueInRange();
}
}
Here’s how it will look in the Unity Inspector when attached to a GameObject:
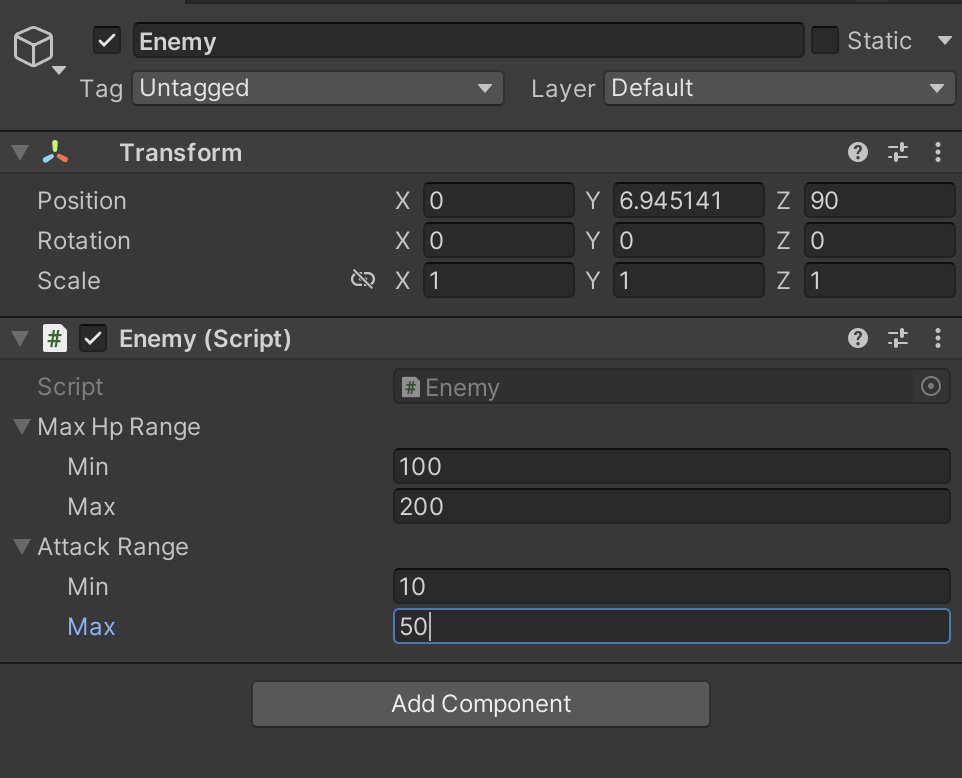
The maximum HP and attack power of each enemy are randomly determined by this script.
Since you no longer have to manage separate fields for Min and Max, the code remains clean and simple.
The Utility Class Can Handle More than Just Integers
As you might have already guessed, this method works with other numeric types as well!
Here’s an example for float types:
using System;
using UnityEngine;
[Serializable]
public class FloatRange
{
[SerializeField]
private float _min;
public float Min
{
get { return _min; }
set { _min = value; }
}
[SerializeField]
private float _max;
public float Max
{
get { return _max; }
set { _max = value; }
}
public float GetRandomValueInRange()
{
return UnityEngine.Random.Range(Min, Max);
}
}
Unlike the integer version, both the Min and Max values are included when working with floats. So if Min is 3.5f and Max is 10.0f, the generated number will be between 3.5f <= x <= 10.0f
.
Please note that the criteria for inclusion/exclusion in the UnityEngine.Random.Range
function are different for integers and decimals!
Lastly
I wrote this post to quickly share a small utility that can make random number generation in Unity a little easier.
It might be too simple for those who are experienced with Unity, but I hope it can be helpful to beginners who are just starting their journey in Unity game development.
In the future, I plan to share more of my custom utilities on this blog, so feel free to check back for updates!